Comprehensive Guide to C Programming: History and Techniques
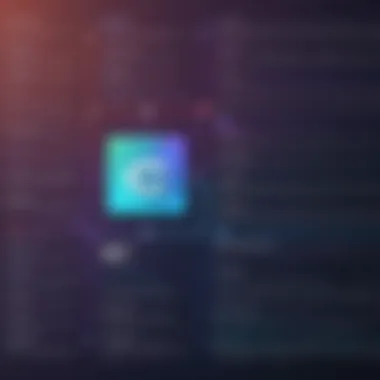
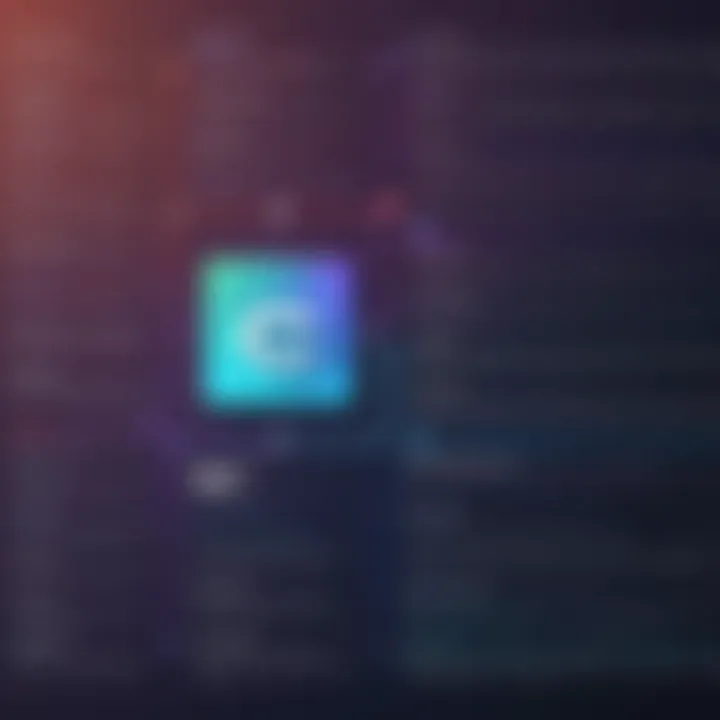
Intro
C programming holds a pivotal position in the tech realm, acting as a bedrock for many programming languages that followed. The journey into C is not merely a stroll through syntactical rules but an intricate exploration of its historical significance, its architectural elegance, and its applications that still resonate in modern computing. In today's ever-evolving tech landscape, understanding C is not just an academic venture; it’s essential for anyone looking to grasp more sophisticated languages or concepts in the realm of computer science.
One cannot underestimate the impact that C has had since its inception. Born in the early 1970s, and developed by Dennis Ritchie at Bell Labs, this language was designed to write system software and application software. What started as a tool for sophisticated computing quickly became the cornerstone for languages like C++, Java, and Python.
As we navigate through this piece, readers will discover the following:
- The rich historical context of C, highlighting milestones that have influenced its development.
- Core structures and syntax which form the basis of C programming and are indispensable knowledge for programmers.
- Real-world applications, showing how C is utilized in fields ranging from embedded systems to operating systems.
- Best practices and pitfalls to avoid, ensuring that one can write efficient, error-free code.
- Advanced techniques that foster a deeper understanding and usage of C, elevating a programmer’s skill set.
Ultimately, this exploration aims to bridge the gap between novice enthusiasts and experienced developers, illuminating the relevance of C in today's tech-driven world. Let’s embark on this journey into a language that, despite its age, remains cutting-edge in its influence.
"C is quirky, flawed, and an enormous success." – Dennis Ritchie
As we delve deeper into C programming, understanding its nuances will not only equip programmers with essential skills but also prepare them for the future of computing.
Foreword to Programming
C programming lays the groundwork for understanding numerous programming languages that followed. It is often referred to as a middle-level language since it combines features of both high-level and low-level programming. Thus, it enables developers to manage hardware resources while maintaining high-level abstractions for ease of use.
In today’s world, knowing C is more than a useful skill; it’s somewhat of a rite of passage for any aspiring programmer. Once you dip your toes into C, you find that it teaches you how computers work under the hood. It’s often the case that learning C helps programmers grasp concepts like memory management, pointers, and function pointers—all essential topics.
Moreover, C has had staying power in technology because of its efficiency. It’s notorious for being a language that gives you control over system resources while still being fairly clean in syntax, especially when compared to assembly. So, it strikes a balance that many developers appreciate. The bottom line is, if you aim to become proficient, be it for systems-level programming or even entering a field like game development, the foundation C provides is irreplaceable.
Historical Background
C was developed in the early 1970s at Bell Labs by Dennis Ritchie as an evolution of the B language. It was crafted to facilitate the development of the UNIX operating system. This early association with UNIX introduced C to a broader audience, and it wasn't long before it became a common indicator of programming prowess.
As the years passed, C underwent various modifications. The first standardized version, known as ANSI C, emerged in 1989, solidifying its status as a cornerstone of programming languages. This standard further enhanced its usability across different platforms and set the stage for the future developments in C and its descendants.
Evolution Over the Years
Since its inception, C has seen multiple iterations and has remained relevant through continual adaptation. Fresh improvements, like the C99 standard, introduced new features such as inline functions, variable-length arrays, and support for complex data types. These enhancements made it appealing not just for systems programming but also for application development.
C’s influence extended beyond its own realm; languages such as C++ and Objective-C emerged, borrowing features and ideas from the language. The introduction of C++ added the concept of objects, a significant shift that influenced modern programming paradigms. Today, many languages still retain C's syntax and structure, proving that once C made its mark, it had lasting implications.
C’s evolution also stirred discussions in programming circles about its relevance. Even as new languages arise, like Python and Rust, which advocate different philosophies, the unfinished business with C continues. Its efficiency, flexibility, and robustness keep it a staple in the toolkit of many developers.
"C programming teaches you how to think like a computer scientist, offering tools that foster problem-solving skills effectively."
In summary, the story of C programming is a narrative interwoven with technological milestones. Understanding its historical context and evolution fuels our appreciation and mastery of the language.
Core Features of Language
The C programming language is often praised for its core features, which set the stage for its ongoing usage and relevance in computing. Understanding these features is essential for both new learners and experienced developers. The core characteristics of C not only support coding efficiency but also enhance programmability in diverse applications. This section dives into two of the most notable features: simplicity and efficiency, alongside portability and flexibility.
Simplicity and Efficiency
C programming is known for its straightforward syntax, which allows developers to write robust code with minimal fuss. This simplicity is largely attributed to its uniformity – it possesses a small set of keywords and constructs. The efficiency of the C language is another critical aspect. Programs written in C can execute quickly, making it a preferred choice in system-level programming. Developers can manage memory directly, which not only allows for more efficient use of resources but also greater control over how applications run.
Some benefits of this simplicity and efficiency include:
- Reduced learning curve: New programmers can grasp the foundational concepts without getting bogged down by unnecessarily complex syntax.
- Speed: With its ability to produce efficient machine code, C is widely adopted in embedded systems, network drivers, and other performance-critical tasks.
- Control: Programmers have the liberty to optimize their applications, thanks to the direct interaction with memory and hardware.
"Simplicity is the ultimate sophistication." - Leonardo da Vinci
This ability to maintain simplicity while maximizing efficiency is a hallmark of C that many other languages aspire to replicate. It’s a calculator's dream where you have a few buttons but can perform complex equations seamlessly.
Portability and Flexibility
Portability is the defining trait that allows C code to translate across various platforms with minimal changes. C was designed with portability in mind, meaning that code written on one machine could easily run on another machine with a compatible C compiler. This is vital for developers who want their applications to run on different operating systems without rewriting the entire codebase.
For example, a video game written in C can run on Linux systems, Windows, and even macOS if it adheres to the standard practices of the language. Flexibility also extends to the style of programming, whether procedural or modular. Developers can easily transition between different coding methodologies while staying within the same language.
Key highlights of C's portability and flexibility include:
- Compatibility: C is everywhere, from microcontrollers to supercomputers. If your code runs well on one system, chances are it will run well on others too, barring certain hardware specifics.
- Easy integration: C can be integrated with other languages and systems, fostering a composite ecosystem for software development.
- Adaptable: Whether you are building a small utility or a large application, C’s flexibility accommodates various project scales and objectives.
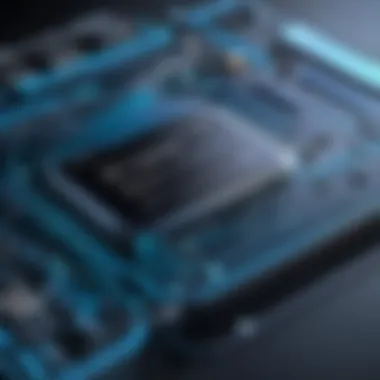
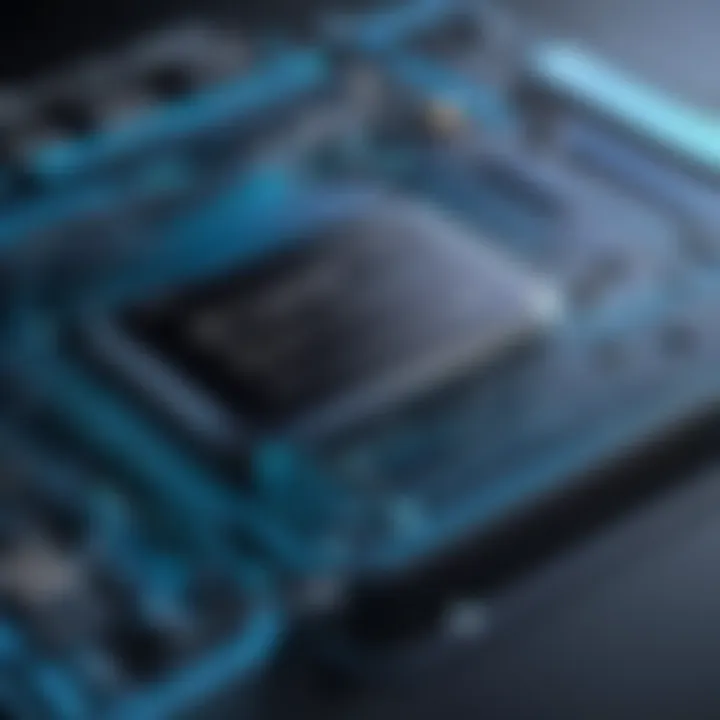
Programming Syntax
C programming syntax lays the groundwork for how programs are structured and how the language is utilized to convey instructions to the computer. Understanding the syntax of C is crucial, as it influences both the functionality of a program and the ease of debugging it. To write effective C code, a programmer must grasp the specific rules governing how statements, expressions, and commands are formed. This understanding facilitates the writing of clear, efficient, and maintainable code.
Basic Structure of a Program
A typical C program comprises several key components. At first glance, it might appear rather simple, but each part has its significance.
- Preprocessor Directives: These begin with a symbol (like ) and are used to include necessary libraries and headers before the compilation of the program begins.
- Main Function: This function, designated as , serves as the entry point for execution. Without it, a C program does not operate.
- Statements: These are the commands that the program executes and can include variable declarations, calculations, and function calls.
- Return Statement: Often, you will find a return statement (e.g., ), signaling the completion of the program.
Consider the following simple example:
In this snippet, you see a clear example of preprocessor directives, the main function, and a return statement, all working in harmony.
Data Types and Variables
In C, data types are essential as they define the kind of data a variable can hold. C has several built-in types:
- int for integers,
- float for floating-point numbers,
- char for characters.
Each type serves a distinct purpose. For instance, variables handle whole numbers, while can manage numerical values with decimals, which might be necessary in calculations involving precision.
Declaring a variable in C is straightforward. For example:
This establishes variables to store an integer for age, a floating-point value for salary, and a character for an initial.
Control Flow Statements
Control flow statements allow programmers to dictate the order in which statements are executed based on certain conditions. This feature is vital for making decisions within a program.
There are several control flow structures in C:
- If Statements: Execute a block of code if a condition is true.
- Switch Statements: Offer a more efficient way to execute different blocks of code based on the value of a variable.
- Loops (For, While): Allow you to repeat a block of code as long as a specified condition holds true.
Here's a practical code snippet demonstrating a simple if statement:
In this example, the program checks if the number is greater than zero and prints an appropriate message. This illustrates how control flow statements empower programmers to create dynamic and responsive applications.
Understanding the syntax of C is essential not just for writing code, but also for debugging and optimizing programs effectively.
With these facets of C programming syntax, programmers build the essential skills to harness the true power of the language. Each component, from how a program is structured to the way data types are managed, plays a pivotal role in effective programming.
Memory Management in
Memory management is a critical aspect of C programming that can make or break the robustness of an application. Understanding how memory operates within a C program provides the foundation for writing efficient, high-performing code. Unlike languages such as Python or Java that handle memory automatically, C requires programmers to take full control over memory allocation and deallocation. This hands-on approach can lead to both powerful capabilities and daunting challenges.
One of the main benefits of effective memory management is performance. When developers can allocate memory selectively and efficiently, they can minimize waste—a common issue in programming. As a bonus, effective management can lead to faster execution times of applications, because less memory overhead means quicker access and manipulation of data.
However, this freedom also comes with significant considerations. The potential pitfalls, such as memory leaks or dangling pointers, can cause applications to crash or behave unpredictably.
"In C, your relationship with memory is like a tightrope walk—balance is essential."
Understanding Pointers
Pointers are arguably one of the most powerful features of C, serving as the backbone of memory management. A pointer is a variable that stores the memory address of another variable. This ability to directly manipulate memory allows for intricate operations like dynamic allocation, array manipulation, and function parameter passing.
At first, the concept of pointers can feel abstract, but grasping their utility is essential. For instance, using pointers can lead to the efficient exchange of large data structures between functions without the overhead of copying data. When you pass a pointer to a function, any changes made to the variable are reflected outside the function as well. This can be particularly beneficial in resource-intensive applications, such as game development or real-time data processing.
One must also tread carefully, as improper use of pointers can lead to serious issues:
- Dangling pointers: Occur when a pointer points to a memory location that has been deallocated.
- Null pointers: These can lead to access violations if dereferenced accidentally.
- Memory leaks: Happen when allocated memory is not properly released, consuming resources unnecessarily.
Dynamic Memory Allocation
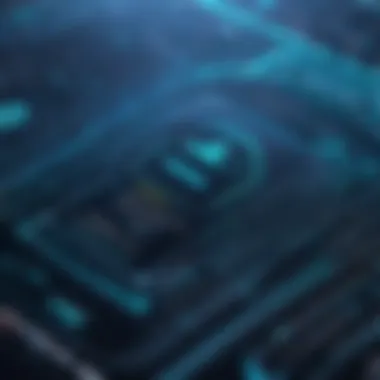
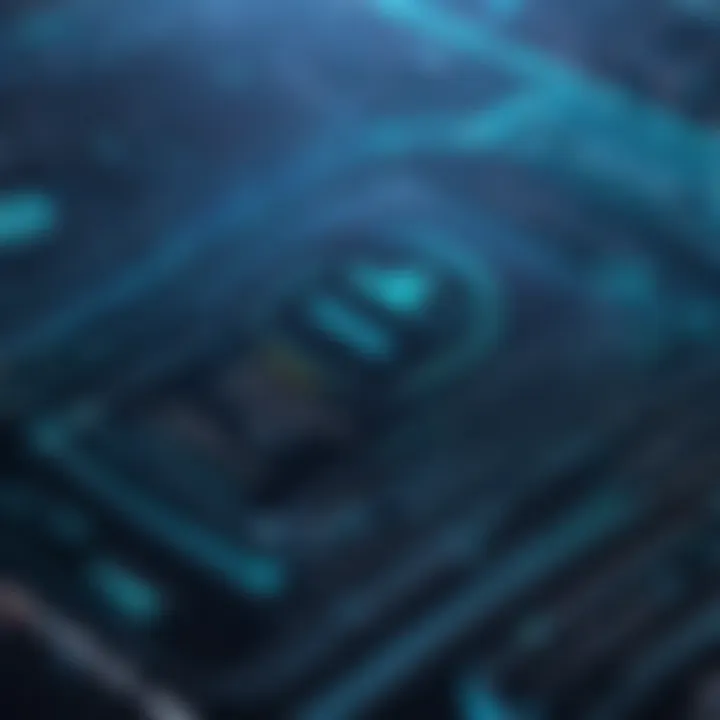
Dynamic memory allocation in C enables users to request memory at runtime, allowing for flexible data structures that can grow or shrink as needed. This is managed primarily through functions like , , , and .
- allocates a specified number of bytes and returns a pointer to the allocated memory.
- allocates memory for an array of elements, initializing all bytes to zero.
- allows you to resize previously allocated memory.
- deallocates previously allocated memory to prevent leaks.
It's essential to check the return values of these functions to ensure that memory was allocated successfully. For instance, if fails, it returns a pointer, which you must handle to avoid dereferencing a null pointer.
Proper dynamic memory management involves understanding how much memory your program requires and promptly freeing it when it's no longer needed. This helps in keeping your applications performant and resource-conscious.
Common Challenges in Programming
C programming, while straightforward in its syntax, poses numerous challenges that can trip up both beginners and seasoned programmers alike. Understanding these challenges is critical, as it equips developers with the tools to navigate the often tricky waters of C. By addressing these common issues, programmers can not only improve their own skills but also advance the overall quality of their projects.
Identifying these challenges allows programmers to take proactive measures, leading to more maintainable and efficient code. It’s about recognizing the pitfalls before they become stumbling blocks. Ultimately, being aware of challenges and knowing how to tackle them lays a solid groundwork for all programming endeavors that may follow in a developer's career.
Debugging Techniques
Debugging is an integral part of the software development life cycle, especially in C programming, where issues can stem from a variety of sources. When bugs creep in – and they will – having a toolbox of debugging techniques becomes invaluable.
- Use of GDB: The GNU Debugger is a powerful tool that allows programmers to analyze and debug their code effectively. You can run your program step-by-step, monitor variable values, and identify where things go awry.
- Print Statements: The classic approach never gets old. Sometimes, a simple statement can reveal volumes about what your program is doing at any given moment. It might not be glamorous, but it’s often effective.
- Static Analysis Tools: Integrating tools such as Splint or Cppcheck can catch potential issues before they turn into runtime errors. They analyze code without executing it, making sure you’re dotting your I’s and crossing your T’s.
- Valgrind: This tool is particularly beneficial for memory management issues. It checks for memory leaks, improper memory accesses, and provides insights that help refine your code.
Debugging isn't just about spotting errors. It's also about understanding the program's flow and logic. Building a methodical approach can help weed out not just the obvious bugs, but also more subtle logical errors that could cause headaches down the line.
"Debugging is being the detective in a crime movie where you are also the murderer." – Filipe Fortes
Handling Errors
Error handling in C might not be as straightforward as in higher-level languages. However, mastering it is essential for creating robust applications. Errors can happen at various stages, such as during compilation, runtime, or even logical errors during execution.
- Return Codes: Many C functions use return codes to indicate success or failure. It’s best practice to check these codes and understand their meaning. Ignoring them is like driving with a broken speedometer; you might be heading for trouble.
- errno and perror: The variable provides a way to communicate error codes when operations fail, especially in functions dealing with system calls. Using helps output a descriptive error message, aiding in quicker debugging.
- Assertions: Utilizing the macro can help you catch unexpected conditions during development. It's a kind of safety net that can alert you to situations that should never occur if everything is working as intended.
- Exception Handling: While C does not have built-in exception handling like C++ or Java, programmers can simulate it using setjmp and longjmp. This allows jumping back to a saved state, albeit with a more complex approach.
In summary, tackling error handling requires diligence and awareness. Being proactive in addressing potential failures sets a solid foundation for software that’s more resilient and user-friendly. Adequate error management not only enhances the longevity of code but also increases the programmer’s credibility.
Best Practices in Programming
Programming in C is not simply about writing lines of code; it's about writing code that other programmers can easily understand, maintain, and optimize. In this section, we will explore best practices that, when adopted, can enhance the quality of your C programs, making them both effective and efficient.
The foundation of any robust software solution lies in its readability and maintainability. Following best practices helps developers avoid common pitfalls that can lead to bugs, inefficiencies, or unnecessary complexities. Consequently, investing the time to write clean code translates into long-term gains in productivity and team collaboration.
Writing Readable Code
Endeavoring to write readable code is akin to refining your craft. It involves organizing your work in a way that is easily navigable. Readable code doesn’t just cater to your own understanding; it embraces future developers who may interact with it. Here are some key elements to keep in mind:
- Consistent Naming Conventions: Use meaningful names for variables and functions. Instead of a generic name like , use . This small shift tells a story about your data, making it clearer what represents.
- Indentation and Formatting: Adhering to a consistent indentation style significantly aids in understanding the structure of your code. Group related lines together clearly, and use whitespace effectively to separate logical sections.
- Modular Design: Break down code into small functions that carry out distinct tasks. Each function can then be understood independently, but together, they create a comprehensive solution. This modular approach not only boosts clarity but also improves code reusability.
By adhering to these principles, your code can transform from a tangled web into a clear roadmap. Here’s a small code snippet showcasing a readable structure:
Effective Commenting Strategies
When it comes to commenting, think of it as benevolent guidance for anyone who might read your code, including your future self. Comments should preserve the intent behind your coding decisions, offering insights that aren't immediately clear from the code itself. Here are some best practices:
- Comment Why, Not What: Clearly articulate the rationale behind your coding decisions rather than describing what the code does. This provides valuable context for future reviews.
- Avoid Over-Committing: Too much commentary can bog down readability. Effective comments are succinct and to the point, adding clarity rather than clutter.
- Keep Comments Updated: As your code evolves, so should your comments. Outdated remarks can lead to confusion and misunderstandings.
"A misunderstood comment could be worse than no comment at all."
By blending readable code with thoughtful commenting, you greatly enhance the overall quality of your C programs. This spirited approach not only benefits you but also your colleagues, ensuring smooth collaboration across diverse teams. In the tech domain, where competence is prized, mastering these practices can set you apart in your programming journey.
Applications of Programming
C programming remains a pivotal force in the computing world, shaping the landscape of software development across various domains. Its widespread applications unveil the significance of C language, portraying it not just as a learning tool but as a powerful engine driving many important technological advancements. From system software development to innovative embedded systems, C demonstrates its unique ability to serve both foundational and complex requirements. This section delves into two primary applications: system programming and embedded systems, both of which showcase C's versatility and enduring relevance.
System Programming
System programming is like the backbone of computing, where software operates very close to the hardware. C thrives in this arena because it offers direct access to memory and low-level system functionality, making it an ideal choice for creating operating systems, device drivers, and system utilities. Operating systems such as Unix and Linux are crafted largely in C, benefiting from its efficiency and performance.
Some of the specific reasons for C's dominance in this domain include:
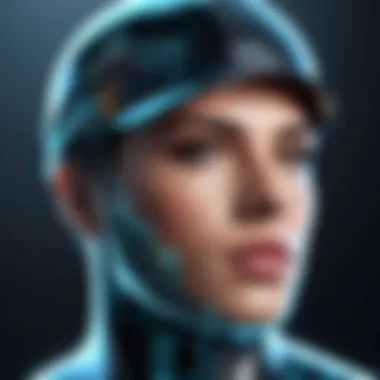
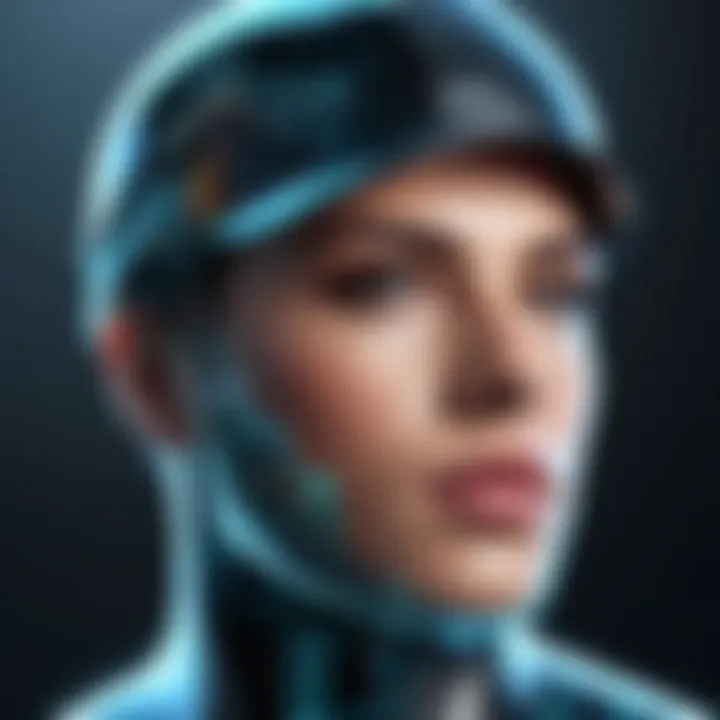
- Performance and Control: C enables programmers to write code that is highly efficient, utilizing system resources judiciously, which is crucial for system software.
- Direct Hardware Manipulation: System programmers often need to manipulate device-specific registers directly. C's pointer arithmetic facilitates these operations effortlessly.
- Portability: While C is a low-level language, well-structured C code can be compiled on numerous platforms with minimal modifications, enhancing its usability across diverse systems.
For instance, whenever a computer boots up, the core functions of the operating system must kick in promptly. C, with its clear syntax and ability to communicate directly with hardware, manages these tasks. This ability underscores how vital C is in system programming, where every millisecond counts, and efficiency is key.
Embedded Systems
Embedded systems are everywhere, from microwaves and washing machines to sophisticated automotive controls. C plays a starring role in their development due to its balance of efficiency and abstraction.
- Real-Time Performance: Many embedded applications require deterministic behavior. C gives developers the capability to meet timing constraints effectively without a lot of overhead.
- Resource Management: C’s efficiency allows programmers to take full advantage of limited hardware resources, making it the go-to choice for low-power and low-cost devices.
- Component Interaction: C can facilitate communication between hardware components efficiently. This is essential in embedded systems where different modules must work in harmony.
A classic example is the use of C in automotive systems for engine control units (ECUs). These systems require real-time response to sensor inputs to modulate fuel injection and ignition timing. Using C in these scenarios allows for precise control and rapid computations, thus enhancing vehicle performance.
"C is not just a programming language; it’s a bridge to the hardware."
Both applications emphasize the ability of C programming to not only operate close to the metal but also provide a platform for building scalable and complex systems. The more you delve into the world of C, the more you discover its foundational role in technology today. Its applications are essential, proving that while new languages may come and go, the impact of C will likely endure long into the future.
The Impact of on Modern Languages
C programming is often regarded as the backbone of modern computing languages. Its influence stretches far beyond its own boundaries, shaping the development of numerous programming languages that followed. This section will delve into how C has left its mark on both C++ and Java, two prominent languages in the programming landscape. We'll explore its legacy in software development, highlighting the benefits and reasoning behind its lasting relevance.
Influence on ++ and Java
C++ and Java are two of the most widely utilized programming languages today, and their roots can be traced back to C. Understanding this influence is crucial for grasping the broader narrative of programming evolution.
- Object-Oriented Features: C++ introduced object-oriented programming (OOP) principles while maintaining C’s efficiency and performance. Features like classes and inheritance allow developers to create structured programs that could scale efficiently. Java, too, built on these principles, introducing its own OOP features while maintaining a syntax that resonates with C.
- Syntax Familiarity: Anyone who has written C will find comfort when transitioning to C++ or Java. The basic syntax is largely the same, making it easier for programmers to adapt without needing to start from scratch.
- Memory Management: Both C++ and Java have added layers to memory management to enhance security and ease. However, they owe their foundational concepts of pointers and memory allocation to C's straightforward approach.
"C has laid the groundwork for many of today’s most vital programming languages—an enduring legacy that continues to shape the tech sphere."
Legacy of in Software Development
The legacy of C in software development is evident in various domains, showcasing its robust principles and adaptability. This foundation paved the way for advancements in how we perceive software construction.
- System-Level Programming: Many operating systems, like UNIX, rely on C for core functionality. The language provides the necessary tools to interact with hardware efficiently, resulting in powerful system-level software that remains relevant.
- Cross-Platform Applications: C is known for its portability. Applications designed in C can often be compiled and run across multiple platforms with minimal adjustments, an important feature in an increasingly diverse technology landscape.
- Performance Optimization: As a lower-level language, C offers direct access to machine-level resources, leading to better performance. This aspect continues to be pivotal for developers working on high-performance applications, particularly in gaming or real-time systems where speed matters.
Programming in the Future
C programming has stood the test of time. Its influence permeates through layers of newer languages and technologies. But as we look ahead, the future of C programming rests not only on its legacy but also on the emerging applications and trends that shape how we write and think about code. The landscape of programming is always shifting, and understanding these changes will ensure that C remains relevant.
Emerging Trends
As technology evolves, several trends begin to surface within the C programming ecosystem. For one, system-level programming is experiencing a rebound in popularity. As more developers focus on performance and efficiency, C provides a way to interact directly with hardware, helping to optimize applications.
- Increased Concurrency: Concurrent programming is on the rise. Libraries such as OpenMP and Pthreads allow C programmers to leverage multi-core processors, enabling richer, faster applications.
- Integration with Modern Tools: Tools such as Docker can now encapsulate C applications, making deployment smoother. This trend simplifies the setup of development environments.
- Focus on Security: With rising cyber threats, there’s a palpable shift towards writing secure code. Initiatives like OWASP and various static analysis tools have started to integrate C programming guidelines into their frameworks.
"The only thing worse than being blind is having sight but no vision." – Helen Keller
Continued Relevance in Academia
In the academic realm, C programming remains a staple in computer science curricula across the globe. There are compelling reasons why this will not change anytime soon.
- Foundation for Learning: C serves as an excellent introduction to programming concepts. It equips students with a solid understanding of how computers work at a fundamental level.
- Engagement with Advanced Topics: Advanced fields such as systems programming, embedded systems, and compiler design often begin with a strong grasp of C.
- Research Applications: New algorithms and theoretical models often rely on C for implementation due to its efficiency. This research is prevalent in areas like artificial intelligence and machine learning, requiring low-level operations.
Culmination
In wrapping up a discussion about C programming, it’s vital to realize how this language remains a linchpin in tech. The very essence of C programming serves not just as a foundational skill but a crucial piece of the puzzle for aspiring developers and seasoned professionals alike.
When we contemplate the significance of the insights derived from this article, we find ourselves echoing several specific elements. Firstly, C's simplicity is often deceptive; its capability for performance optimization is a boon for intricate system programming. Moreover, understanding memory management in C - through pointers and dynamic allocation - equips programmers with a level of control rarely found in newer languages.
The benefits are clear. C programming fosters a deeper understanding of how computers operate behind the scenes. The meticulous attention to detail in memory allocation or error handling translates into a more robust programming practice that can elevate any software project. C's continuous relevance in academia ensures that the next generation of developers will be prepped to tackle challenges in emerging fields.
This holistic exploration emphasizes not just the benefits of mastering C, but also its consideration as a cornerstone of software development. As we transition into environments that prioritize rapid development cycles, understanding C's foundational practices will guide future endeavors in any programming language.
"A solid grasp of C can be a stepping stone to unlocking the intricacies of other languages and systems, making it an evergreen skill in a constantly changing technological landscape."
Recap of Key Points
To distill our journey through C programming, we can highlight several key points that underpin its importance:
- Historical Context: Recognizing C's rich history helps contextualize its influence on newer languages.
- Core Features: C’s simplicity and efficiency remain central, demonstrating a clear path to performance gains.
- Memory Management: An understanding of pointers and dynamic memory allocation prepares developers for nuanced programming challenges.
- Best Practices: Emphasizing readability and effective commenting creates foundational disciplines in programming.
- Applications: Whether in system programming or in embedded systems, C prevails in crucial fields, reaffirming its ongoing relevance.
Final Thoughts on Programming
As we reflect on C programming, it stands as a testament to the strength of foundational languages in the ever-evolving landscape of technology. Mastering C isn't just about learning syntax or understanding memory management; it’s an exercise in problem-solving and a gateway into higher-order thinking about software architecture.
The future of programming will undoubtedly witness shifts towards automation and higher-level abstractions, yet C's methodologies will echo within many of these trends. The legacies of C's influence on contemporary languages like Python and JavaScript signal that the historical significance will not fade away. Instead, it transforms, demanding new skills and adaptations, all while anchoring us to the principles that underlie software development. Truly, a diligent programmer’s toolkit is incomplete without the wisdom and knowledge derived from C.