Thinking Like a Coder: A Guide to Problem-Solving
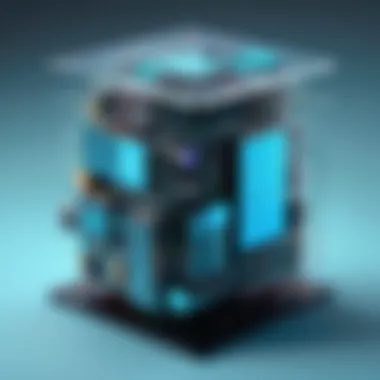
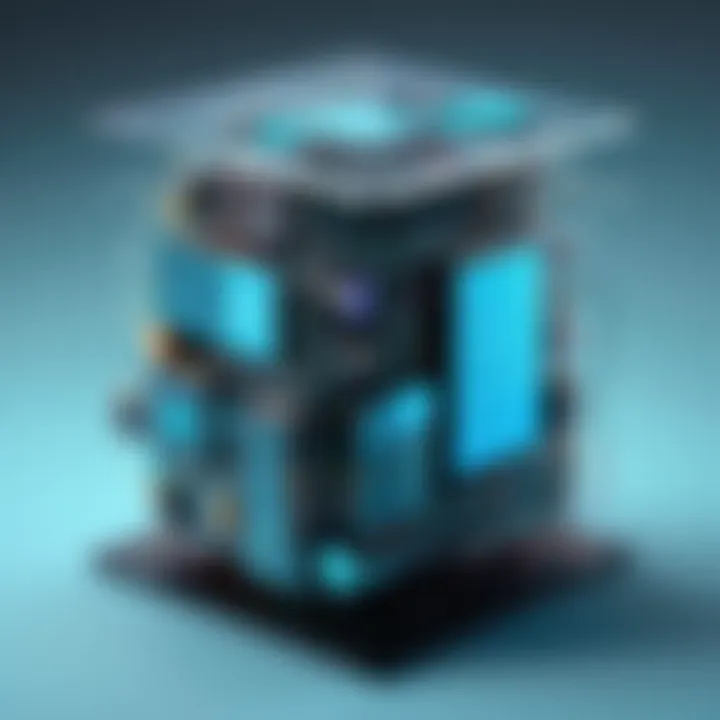
Intro
In the world of coding, thinking isn't just a processāit's a vital skill that shapes how a programmer approaches problems and crafts solutions. The ancient adage, "measure twice, cut once," rings true here; yet in coding, itās vital to think twice before jumping into the code.
Understanding coding isn't merely about grasping syntax or employing the latest libraries. Rather, itās about mastering a mindset that allows for a clearer, more logical way of thinking. This guide seeks to illuminate the pathways to developing this essential coder's mindset, empowering both newbies and seasoned programmers alike.
Designing software or web applications can often feel like navigating through a dense forest without a map. A programmer equipped with a systematic approach can find their way around challenges with ease, rather than thumping into the nearest tree.
Key Points We'll Explore
- The importance of logical reasoning in programming.
- Systematic approaches that can simplify complex challenges.
- Techniques for fostering a deeper engagement with coding practices.
By delving into these facets, youāll uncover not only how to think like a coder but also why such a perspective is crucial in todayās tech landscape. Let's get started.
Intro to Coding Mentality
When we talk about coding, the conversation often revolves around languages, syntax, and frameworks. However, the crux of excelling in programming lies not just in writing code, but in adopting a mindset that allows one to think logically and systematically. This is where the concept of the "coding mentality" comes into play. Understanding what it means to think like a coder is key to unlocking greater problem-solving skills and fostering innovation within technology.
Understanding the Programmer's Mindset
The programmer's mindset goes beyond mere technical knowledge. It is a way of approaching challenges that promotes deep engagement with the complexity of problems. At its core, this mindset involves the following elements:
- Curiosity: An innate desire to explore how things work. Coders often find themselves dissecting problems or products to understand the underlying principles that govern their functionality.
- Analytical Skills: A thinker who enjoys breaking down situations into manageable parts will find themselves at an advantage. This means analyzing a problem component by component, assessing the impact of each, and ultimately forming a holistic understanding.
- Creativity: Contrary to the stereotype, coding is not purely logical; creativity plays a vital role. It's about envisioning multiple solutions for a single problem and weighing the pros and cons of each.
In practical terms, when faced with daily coding dilemmas, a proficient coder first examines the issue, sketches out potential methods to tackle it, and then chooses a path forward, not simply based on convention but on informed intuition born from experience.
The Importance of Logic in Programming
Logic serves as the backbone of programmingāit is the skeleton upon which successful code is built. Without a solid grasp of logic, even the most well-structured code can lead to unexpected results and bugs. Here are some key considerations regarding logic in coding:
- Algorithm Design: Logic is fundamental when developing algorithms. An algorithm is simply a step-by-step procedure to solve a problem, and crafting efficient algorithms requires clear logical thought.
- Debugging: Logic plays its part in debugging too. Recognizing the logical flow of the program helps identify where things diverge from the expected path, making it easier to locate and fix bugs.
- Conditional Statements: The use of conditions in codingāsuch as if-else statementsārests entirely on logical structures. A coderās ability to predict outcomes based on specific inputs relies on clear logic.
To sum it up, embracing a coding mentality fosters a powerful combination of logic, creativity, and systematic thinking. By cultivating these aspects, one doesn't merely write code; one becomes adept at solving complex, multifaceted problems that reside at the heart of software development.
"The beautiful thing about learning is that no one can take it away from you." - B.B. King
In the realm of coding, this encapsulates the essence: developing a coder's mentality is not merely an academic exercise, but a lifelong journey toward mastering the art of problem-solving.
Fundamentals of Logical Thinking
Logical thinking is the backbone of effective coding. It's not just about knowing how to write lines of code; it's about understanding the how and why behind solving problems. In programming, having a strong foundation in logic aids greatly in devising solutions that are efficient and adaptable. The process can become daunting; however, grasping these fundamentals paves a clearer path toward cultivating a coder's mindset.
The Role of Algorithms in Problem Solving
Defining Algorithms
An algorithm is essentially a step-by-step procedure for solving a problem or accomplishing a task. Imagine a recipe for baking a cake; you follow a series of steps to transform raw ingredients into a delightful dessert. Algorithms are much like recipes in that they provide structure and guidance.
A key characteristic of defining algorithms is their clarity and precision. It's this clarity that makes them a popular choice in programming, acting as a common language among coders. The unique feature of algorithms is their versatility, applicable across different programming languages and paradigms. They come with both advantages, like efficiency in problem-solving, and disadvantages, such as complexity in design for very intricate problems.
Types of Algorithms
When it comes to types of algorithms, there is a multitude to choose from, each designed for specific tasks. Common categories include sorting algorithms, like QuickSort and MergeSort, as well as searching algorithms, like binary search. The key trait here is specializationādifferent algorithms serve different purposes.
Types of algorithms are beneficial as they can be utilized depending on the situation at hand. For example, if you're faced with a large dataset, selecting the right sorting algorithm can significantly cut down processing time. Their unique feature is the algorithm's adaptability to various data structures, although this can lead to overwhelming options for beginners.
Algorithm Complexity
Algorithm complexity refers to the measurement of the performance of an algorithm in terms of time and space. Simply put, it's about how an algorithm's run-time or memory usage grows as the input size increases. This idea can be likened to running a marathon; the more miles you add, the longer it takes to finish.
A critical aspect of algorithm complexity is its efficiency analysis. It's a valuable component because understanding how an algorithm behaves with increasing input is essential. The unique feature here is its focus on scalability, which allows programmers to make informed decisions about what algorithm to implement as the program grows. Nonetheless, there can be disadvantages, such as the steep learning curve associated with mastering complexity analysis.
Breaking Down Complex Problems
Decomposition Techniques
Decomposition techniques involve breaking a large problem into smaller, manageable parts. This method can be highly efficient, much like dividing a hefty task into bite-sized pieces you can handle one at a time. The critical characteristic of decomposition is that it makes tackling problems less overwhelming, forcing the thinker to clarify different elements of the issue at hand.
This technique is beneficial because it helps in pinpointing exactly where to focus your efforts when coding. The unique feature is its emphasis on clarity and organization, although it could lead to unnecessary complexity if not carefully structured or if too many subdivisions take place.
Sub-problems and Their Importance
Sub-problems play a pivotal role in problem-solving within coding. These smaller issues draw from the larger problem and foster deeper understanding when working towards solutions. Their significance lies in their potential to provide insights into the overall challenge, elucidating paths and connections that may have been initially overlooked.
Recognizing sub-problems as individual but interconnected elements of a larger equation makes them a beneficial focus area. The unique feature of sub-problems is their reliance on patterns from earlier solutions, which aids in problem-solving efficiency. However, one must be cautious: focusing too heavily on sub-problems may lead to losing sight of the broader goal.
Developing Effective Coding Strategies
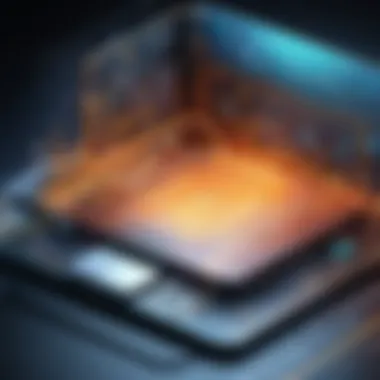
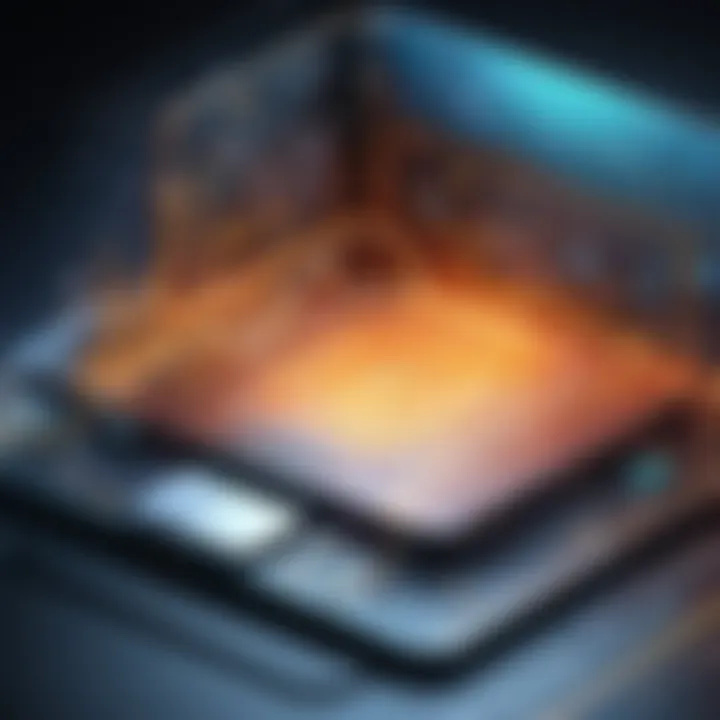
In the realm of coding, understanding effective strategies is paramount. Itās not just about slinging lines of code; it's about crafting a systematic plan to tackle complex problems. When you get a grip on these strategies, you can elevate your coding prowess and develop solutions that are not just functional but also efficient and elegant.
Effective coding strategies allow you to organize your thoughts, reduce errors, and improve collaboration. They often lead to cleaner, more maintainable code that can easily be passed on to others, especially in team environments. By honing your approach, you can optimize your workflow and maximize both your productivity and the quality of the final product.
Understanding Data Structures
A solid grasp of data structures is essential for any coder. They determine how data is organized and managed, playing a significant role in the overall efficiency of your code. Let's dig into a few key data structures: arrays, linked lists, and hash tables.
Arrays
Arrays are one of the most fundamental data structures in programming. They allow you to store multiple values in a single variable, which can significantly simplify code and improve performance. The beauty of arrays lies in their simplicity; they are indexed collections, making the retrieval of elements efficient. However, one must be aware that arrays have a fixed size, meaning that once created, their size cannot change without creating a new array. This can sometimes be a limitation, especially if you don't know how much data you'll need to store in advance.
- Key Characteristics:
- Advantages:
- Disadvantages:
- Fixed size
- Indexed access
- Contiguous memory allocation
- Fast access time
- Memory efficiency for known fixed sizes
- Resizing can be costly
- Inefficient when data size is dynamic
Linked Lists
Linked lists bring a different flavor to data organization. Unlike arrays, they are dynamic and can grow or shrink as needed. Each element, or "node," contains data and a pointer to the next node in the sequence. This makes inserting or deleting elements a breeze, as you donāt have to deal with shifting other elements like you would with arrays.
- Key Characteristics:
- Advantages:
- Disadvantages:
- Dynamic size
- Nodes containing data and pointers
- Efficient insertions/deletions
- No need for pre-allocation
- More memory overhead due to pointers
- Slower access time compared to arrays
Hash Tables
Hash tables take a different approach to how data is stored and accessed. They are designed to provide fast data retrieval using a key-value pair system. They utilize a hash function to compute an index into an array of buckets, from which the desired value can be found. Hash tables are revered for their average-case time complexity for searches, which is O(1).
- Key Characteristics:
- Advantages:
- Disadvantages:
- Key-value pairs
- Fast average-case lookup
- Very fast data retrieval
- Flexible sizing (can handle varying amounts of data more gracefully)
- Potential for collisions
- Memory consumption can be high if not managed well
Choosing the Right Tools for Coding
Just like a painter needs the right brushes and colors, a coder must select the right tools. The tools you use can significantly shape the coding experience and the quality of your output. Here, weāll explore the importance of IDEs, text editors, and programming languages.
IDEs and Text Editors
Integrated Development Environments (IDEs) and text editors vary widely from one another, but both serve as fundamental tools in a coderās toolkit. IDEs like Visual Studio or IntelliJ IDEA offer a plethora of built-in featuresādebugging tools, build automation, and version control capabilities all wrapped in one package.
- Key Characteristics:
- Advantages:
- Disadvantages:
- Feature-rich
- Integrated debugging tools
- Streamlined workflows
- Easier debugging and project management
- Can be resource-heavy
- May be overwhelming for beginners
Conversely, text editors such as Sublime Text or Visual Studio Code offer lightweight alternatives. They often require plugins to reach the functionality of an IDE, allowing for a more customizable approach but might necessitate some manual setup for complex projects.
Programming Languages and Their Applications
Programming languages act as the medium through which you express your ideas in code. Each one comes with its syntactical nuances, strengths, and ideal use cases. Languages like Python allow for rapid prototyping and are user-friendly, making them popular among beginners, while languages like C++ offer performance advantages.
- Key Characteristics:
- Advantages:
- Disadvantages:
- Syntax and semantics unique to each language
- Various community, libraries, and frameworks available
- Flexibility in project scope and execution time
- Large support communities
- Each language comes with its own learning curve
- Not all languages are suited for every task
The choice of tools and data structures you wield shapes how effective and efficient your coding journey will be. As you conquer these aspects, you'll find your coding practice transforming into a well-oiled machine, ready to tackle whatever challenges come your way.
Approaching Problems Systematically
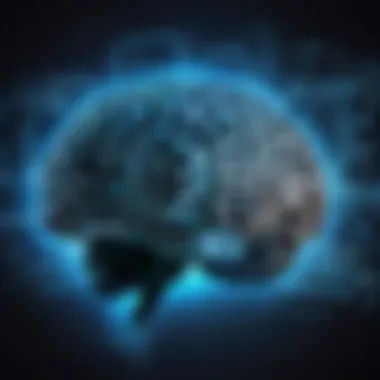
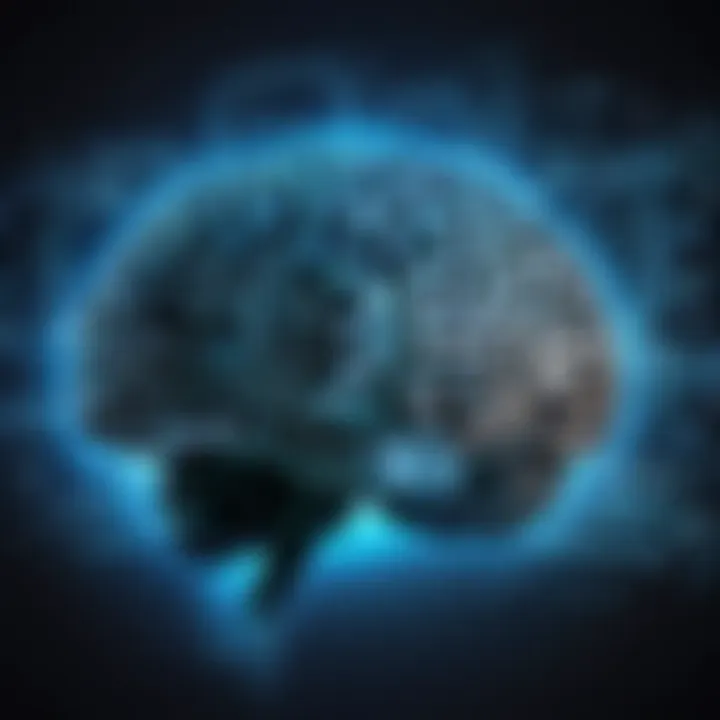
When it comes to coding, the ability to approach problems in a structured manner is crucialāit's the backbone of effective solutions. You can't just dive in headfirst and hope for the best. Instead, a systematic approach lets you break down complex issues into manageable pieces. This not only makes the coding process smoother but also increases your chances of success.
By adopting systematic methods, you enhance your problem-solving capabilities. It involves various practices and frameworks that help in knocking down your challenges step-by-step. Relying on these processes can boost your confidence when tackling new issues. Let's explore the debugging and testing processes, which play significant roles in this approach.
The Debugging Process
Debugging is more than just fixing bugs; itās a comprehensive method of understanding where things might be going a bit sideways in your code.
Identifying Bugs
Identifying bugs is a fundamental aspect of debugging. Think of it as detectives gathering clues. Every time your code doesnāt behave as expected, you are effectively investigating a crime scene. This phase is all about harnessing precision. The key characteristic is its methodical approachāpinpointing the exact location of the issue is paramount. Why is this beneficial? Because without nailing down the bug, you're shooting in the dark.
One unique feature is the capacity to analyze log files and error messages, which serve as breadcrumbs leading you back to where your code went astray. The advantage here is clarity: you get a clear view of the functionality gone wrong. However, the downside could be time consumption. Sometimes, hunting for those bugs feels like searching for a needle in a haystack.
Debugging Techniques
Once you nail the bug down, you need effective debugging techniques at your disposal. This is where a toolbox of methods comes into play. These techniques can range from simple print statements to more complex processes like using debuggers. The hallmark of a good debugging approach is adaptability; choosing the right technique based on the situation is key. This makes it a beneficial choice throughout coding endeavors.
What sets these techniques apart is their potential to create snapshots of the program execution. It allows you to step through the code one line at a time and analyze behaviors. The advantage is thorough understanding; but if done excessively, you might end up over-complicating what could have been a straightforward fix.
Testing Your Code
Testing is not an afterthought; it's an integral part of the coding lifecycle. It legitimizes your codeās functionality by ensuring that it does what it's supposed to do.
Unit Testing
Unit testing allows you to check individual components in isolation, like checking if a light switch works before installing it into the entire electrical system. The key characteristic of unit testing is its ability to identify small-scale issues that, if left unchecked, could snowball into larger problems. Itās a popular choice because it gives you confidence that your individual pieces are performing as expected.
One unique feature is the creation of test cases that define specific scenarios. This focused approach reduces ambiguity in development and can save considerable time down the line. However, if you neglect integrating unit tests early in your project, you might face a formidable pile of corrections later.
Integration Testing
Integration testing takes the components you've validated through unit testing and checks how they all work together. Think of this as rehearsing a concert. Each musician knows their part, but the real test is how well they harmonize when they all play together. The key characteristic of integration testing is its focus on interactions between components, helping to identify breakdowns in collaboration. This is a great way to ensure systems operate smoothly when layered upon each other.
One notable aspect is that you can catch discrepancies that might not show up with unit tests alone. While it makes sure everything collaborates seamlessly, integration testing can become complexālike mixing various ingredients in a pot; it might create unexpected flavors that need additional tweaks.
In summary, approaching problems systematically while leveraging effective debugging and testing practices is integral for coders. It locks in quality and efficiency, helping you to navigate through the maze of coding challenges.
Enhancing Your Coding Efficiency
Efficiency in coding is akin to having a well-oiled machine. In the fast-paced world of programming, where deadlines loom like storm clouds, enhancing your coding efficiency is not just beneficial; it is essential. As technology evolves, the requirement for speed and accuracy becomes even more pressing. Making your coding processes more efficient can lead to improved productivity, maintainability, and ultimately better outcomes. In this article, we focus on specific elements like code reusability and version control systems, which are vital for optimizing efficiency.
Code Reusability
One of the pivotal aspects of coding efficiency is code reusability. The essence of reusability lies in writing code that can be utilized in multiple places or projects without modification. This not only saves time but also reduces the likelihood of bugsābecause if everthingās built once, why build it again?
DRY Principle
The DRY Principle, which stands for "Don't Repeat Yourself," is a cornerstone of code reusability. It advocates for the elimination of redundancy by ensuring that every piece of knowledge should have a single, unambiguous representation within a system.
"The best code is no code at all; if you can avoid duplicating it, you open the door for greater maintainability."
One key characteristic of the DRY Principle is its ability to simplify code management. It facilitates a smoother workflow, especially in team settings where multiple developers might be interacting with the same codebase. A unique feature of this principle is that it not only leads to less code but also makes it easier to implement changes when necessary. However, one downside is that it may sometimes lead to overly abstract code, which can be challenging for newcomers.
Modular Programming
Modular Programming takes the idea of reusability further by breaking a program into smaller, self-contained, interchangeable modules. Each module is designed to accomplish a specific function. This approach simplifies debugging and testing because it narrows down the potential areas where bugs might be hiding.
The key characteristic of modular programming is its emphasis on separation of concerns. By isolating functionalities, you can enhance code clarity and maintainability. A defining feature of this method is that it promotes collaboration, allowing multiple developers to work on different modules simultaneously. On the flip side, developing each module as a fully functional piece can take extra time and effort initially.
Version Control Systems
Version control systems play an irreplaceable role in enhancing coding efficiency. They allow you to track and manage changes to your codebase over time. Instead of fearing mistakes, developers can work more freely, knowing that they can always revert changes if necessary.
What is Version Control?
At its core, version control is a way to manage revisions and changes to documents, specifically code in this context. It involves software that keeps track of every modification to the code in a special repository. This capability is essential not only for debugging but also for collaboration when working with others.
A significant characteristic is that it permits multiple developers to work on the same project without overwriting each otherās changes. It's a remarkable way to ensure that everyone is on the same page. However, the learning curve associated with adopting a new version control system can be a barrier for some.
Popular Systems: Git and SVN
When it comes to version control systems, Git and SVN (Subversion) often take center stage. Both tools allow you to track changes and collaborate effectively, but they come with their own sets of characteristics.
Git stands out for its distributed nature, which means each developer has a complete copy of the repository on their local machine. This makes operations remarkably fast. On the other hand, SVN is centralized, where a single server holds the code and everyone pulls from it. While SVN offers a simpler model, it can introduce bottlenecks as all actions must be performed on the server.
Cultivating a Growth Mindset in Coding
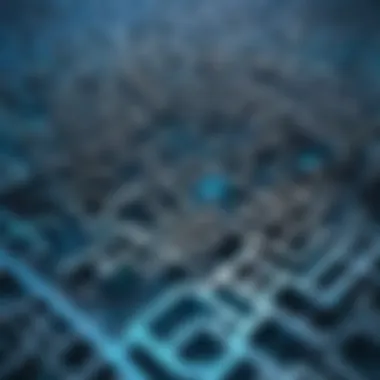
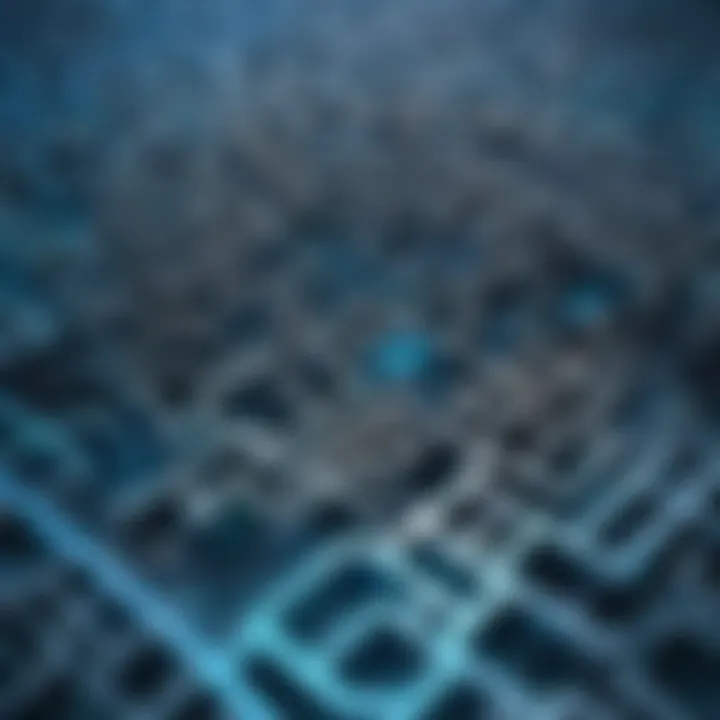
To truly excel in coding, nurturing a growth mindset is essential. This philosophy not only fosters resilience but also shapes the way programmers approach challenges. With technology always evolving, adaptability becomes a key characteristic for anyone looking to keep pace. A growth mindset means viewing challenges as opportunities for growth rather than setbacks. It's about embracing the journey of learning, where mistakes serve as stepping stones rather than roadblocks.
Adopting this psychological framework can have several benefits, such as:
- Enhanced Problem-Solving Skills: Those with a growth mindset tend to engage more deeply with complex issues, allowing them to come up with creative solutions.
- Continuous Improvement: By actively seeking feedback and challenging oneself, programmers can refine their skills over time.
- Increased Motivation: Embracing the idea that abilities can be developed keeps motivation high, even when faced with hurdles.
However, it isnāt a one-size-fits-all approach. Individual experiences play a significant role, and what works wonders for one person might not have the same effect on another. That said, the importance of cultivating a growth mindset cannot be stressed enough in todayās fast-paced tech world.
Learning from Mistakes
Learning from mistakes is a foundational aspect of a growth mindset. In codingāwhere errors are often the norm rather than the exceptionāembracing failures can lead to significant breakthroughs. Instead of treating bugs as a source of frustration, they should be seen as valuable learning experiences. The coder who actively reflects on their mistakes will likely develop a deeper understanding of their craft. This reflection also aids in avoiding similar pitfalls in the future, thus honing oneās skills.
Continuous Learning and Adaptation
Staying Updated with Trends
Staying updated with trends is not just about keeping up with the latest frameworks or programming languages; itās about understanding the direction in which technology is headed. This knowledge is crucial as it can inform a programmerās strategy for their projects. The tech field is notorious for its rapid changes, making it a necessity rather than an option for coders to keep their skills sharp.
One key characteristic of staying updated is its capacity to foster innovation. When programmers engage with trending topics or technologies, they are more likely to encounter fresh ideas and inspiration that can fuel their ongoing work. However, the challenge here is managing the flood of information. Too many trends can lead to confusion or even burnout, which emphasizes the need for selective engagementāfocusing on what's genuinely relevant to oneās work.
Effective Learning Resources
Effective learning resources are vital for sustaining a growth mindset. The web is overflowing with materialsāfrom online courses to coding bootcamps and forums. Varied resources enable individuals to consume information in ways that best fit their learning styles. The availability of platforms like freeCodeCamp, Udemy, or Codecademy allows programmers to broaden their perspectives and dive into new areas of interest.
One decisive characteristic of effective learning resources is accessibility. Many of these platforms offer courses that can be taken at the learner's pace. This flexibility can lead to higher retention rates and better understanding. However, with such a vast selection also comes a potential downside: sifting through the options to find high-quality resources can be time-consuming. Additionally, many resources may not cater to specific, nuanced coding challenges, thereby limiting their effectiveness in certain cases.
By embracing continuous learning and effectively utilizing available resources, programmers are equipped to thrive amid the ever-evolving landscape of technology.
Real-World Application of Coding Thoughts
The world of coding is not just about writing lines of code that perform specific tasks; itās also deeply about applying those thoughts and methodologies to solve real-world problems. This section dives into how coding thought processes translate into practical applications that programmers encounter daily. Understanding how to apply coding principles in real-world situations can enhance problem-solving skills enormously. It fosters creativity and innovation in approaching challenges, turning abstract concepts into tangible outcomes.
Case Studies in Problem Solving
Open Source Contributions
Open source contributions serve as a vibrant canvas where amateur and professional developers alike can paint their skills. A key aspect of this idea is that these contributions encourage collaboration, taking the spirit of coding beyond isolated projects. Developers contribute to established projects, learning from one another while sharing their own expertise. This mutual exchange is prominent in platforms like GitHub and allows anyone to jump in and help in real-time.
The characteristic that makes open source so appealing is its community-driven model, where users are both consumers and creators. Itās a beneficial choice for fostering understanding of complex concepts while giving back to the community. A unique feature of open source is its transparent nature, which allows new developers to view, modify, and learn from the existing codes, but it does come with the downside that not every project receives high-quality contributions. Yet, amidst this noise, the quality projects truly shine.
Real-World Projects
When looking at real-world projects, the application of coding takes on a very practical, result-oriented dimension. Unlike hypothetical exercises, real-world projects require developers to address genuine needs and challenges, enhancing skills and insights. These projects can range from building a small personal app to contributing to a large enterprise system involving complex interactions and data flows.
A notable characteristic of real-world projects is that they often blend business acumen with technical delivery. This makes them a popular choice as they teach developers to think beyond the code itself and understand user needs and business implications. Each project showcases unique features, including stakeholder management and user testing, but the potential downsides can include tight deadlines and shifting requirements. Nevertheless, navigating these constraints can develop resilience and adaptability in coding practices.
Collaboration in Software Development
Working with Teams
In today's tech landscape, collaboration is king. Working with teams highlights the collective power of diverse skills. Itās often said that no one is an island, and in software development, this rings particularly true. When programmers work together, they combine strengths, sharing knowledge and perspectives that help solve problems more efficiently.
The essential characteristic of teamwork is the varied skill sets that individuals bring to the table. This makes teams not only beneficial but also necessary for large-scale projects. The unique feature of team projects lies in the opportunity to specialize while also learning from peers. Challenges can arise, such as conflicts in ideas or differing coding styles, but these moments can lead to stronger solutions if handled properly.
Communication Skills in Coding
The unsung hero in programming is often the communication that goes into it. Strong communication skills are invaluable, as they allow developers to articulate problems, solutions, and complexities involved in coding to diverse audiences, including non-technical stakeholders. In essence, the ability to translate coding jargon into everyday language is a critical aspect of bridging gaps between technical and non-technical teams.
This characteristic of communication in coding is often highlighted as a foundational skill, making it a significant focus for developers aiming for success. Its unique feature is that clear communication often saves time and reduces errors in the development process. However, neglecting this aspect can result in misunderstandings that lead to escalated issues and project delays, exhibiting the need for consistent emphasis on this skill in the coding landscape.
"Code is like humor. When you have to explain it, itās bad." - Cory House
End: The Journey of a Coder's Mind
As we wrap up this exploration into the coding mentality, itās essential to acknowledge that the journey of a coder's mind is nothing short of evolving. The conclusions drawn from understanding the psyche behind coding offer a multifaceted view, not just of the act of programming, but of how we approach problem-solving in varied contexts. This conclusion serves to encapsulate the overarching themes discussed throughout the article, reinforcing the significance of foaming a clear perspective on codingāmore than merely tapping keys, it's about thought processes, adaptability, and logical reasoning.
Reflecting on the Coding Mindset
Reflecting on the coding mindset reveals the core tenets that drive effective programming. Itās like tuning a fine instrument; you want to ensure that every string resonates harmoniously with the others. Coders often shift their focus between the micro and macro aspects of problem-solving. On the micro-level, attention to detail mattersāunderstanding syntax, recognizing bugs, and debugging efficiently. Meanwhile, at the macro-level, the ability to see patterns and frameworks across projects fosters creativity and innovation.
To put it plainly, a coderās mindset isnāt merely about the skills to write code. It's about developing a critical lens that aids in dissecting challenges and discovering pathways to solutions. Often, this reflection leads to a deeper understanding of oneās coding style and preferences, paving the way to formulate a unique approach tailored to personal strengths.
"The coder's journey is a constant cycle of reflection and adaptationāskills that apply far beyond the screen."
When programmers take their time to reflect, they cultivate mindfulness towards their work. This practice encourages them to learn from both successes and failures alike, creating a feedback loop that ultimately sharpens their coding acumen.
Looking Ahead: Future of Coding Practices
Looking toward the future of coding practices, itās clear that technology won't sit still. Skills learned today will continue to adapt and evolve, necessitating an agile mindset among programmers. As artificial intelligence continues gaining traction and automation becomes the norm, a coder will need to be both a learner and an innovator.
In essence, the future hinges on:
- Cross-discipline knowledge: Embracing concepts from fields like data science, machine learning, and cloud computing will enrich a coder's toolkit.
- Community engagement: Participating in forums like Reddit and contributing to open-source projects fosters collaboration and peer learning.
- Embracing new paradigms: Whether it's agile, DevOps, or continuous deployment, coders should stay flexible, adopting methodologies that suit their project's unique needs.
- Continuous professional development: Online courses from platforms like Coursera or resources found on Wikipedia will keep one's knowledge fresh and relevant.
As we navigate these uncharted waters, letās remember: the essence of coding isn't just in writing lines of code; itās in nurturing a mindset geared for adaptability and problem-solving. This approach not only prepares coders for a current landscape but offers unexpected tools for navigating complexities in the future, ensuring that the journey of a coderās mind remains dynamic and impactful.